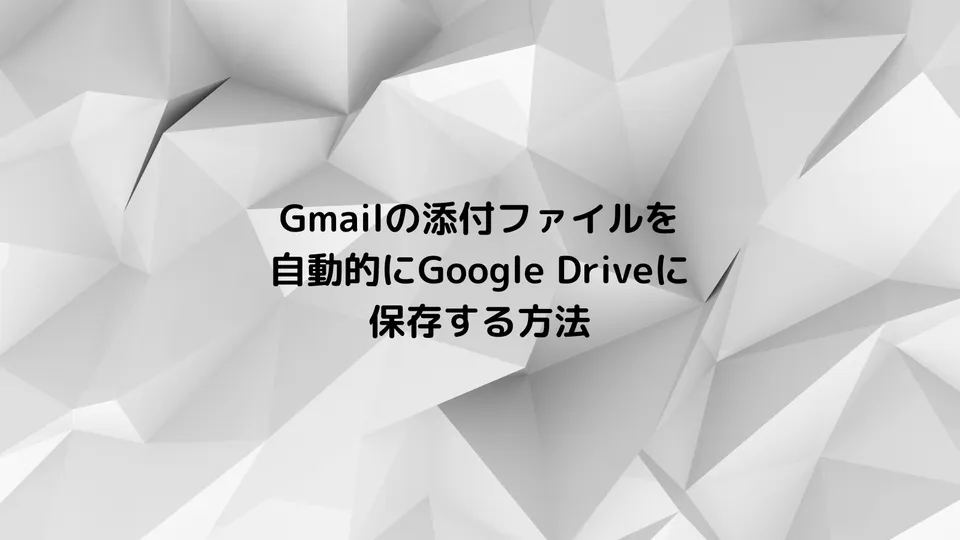
Gmailの添付ファイルを自動的にGoogle Driveに保存する方法|Google Apps Script(GAS)
Gmail のメーリスで時折添付されてくる重要なファイルを忘れずに保存したいけど、いちいちメールから適切なフォルダに保存するのがめんどくさいので、自動で Google Drive に保存するようにしたい、というモチベーションはあるだろう。
Google Apps Script(通常、GAS)でできるらしいのでやってみた。
やろうとしたこと
- Gmail の特定の検索条件に該当するメールの一覧を取得
- 指定した Google Drive のフォルダを参照
- 該当フォルダにすでに同名のファイルがあるかどうかを確認
- 同名ファイルがない場合のみ、メールに添付されているファイルを Google Drive に保存
いくつか先行記事があったので参考にしたが、最新の一日分のみを保存対象とするようにするなどの方法で重複排除をしていた。
しかし、初回は最新の一日分のみならず全件対象にしたいなどいろいろ条件があると思うので、ファイル名で重複を判定するようにした。
こうすることで、どれくらいの頻度で実行しても、重複したファイルは保存されないようになる。
ただし内容の違う同名ファイルは重複とみなされ保存されないので、その可能性だけには留意が必要である。厳密に行こうとするならば、ハッシュ値を取得して比較するなどの処理が必要になるだろう。
あとは、ファイルを見たくなるようなメールはいつも星をつけていたのでその処理を入れているが、扶養な方は既読にするなり、ラベルをつけるなり、好きなようにカスタマイズするとよいだろう。
実際のコード
// Google Driveの該当フォルダのIDを自分で指定してください
const FOLDER_ID = "your_folder_id";
function main() {
// 検索条件を設定
const searchConditions = [
"has:attachment", // 添付ファイルがある
"to:[email protected]", // 特定の宛先に送られた
];
const searchQuery = searchConditions.join(" ");
const searchResults = GmailApp.search(searchQuery);
const messages = GmailApp.getMessagesForThreads(searchResults);
// メールを処理
for (const threadMessages of messages) {
for (const message of threadMessages) {
const attachments = message.getAttachments();
saveAttachmentsToDrive(attachments);
}
// スターを付ける
threadMessages[0].star();
}
}
function saveAttachmentsToDrive(attachments) {
// Googleドライブのフォルダを指定
const storageFolder = DriveApp.getFolderById(FOLDER_ID);
// 添付ファイルを保存
for (const attachment of attachments) {
const filename = attachment.getName();
let existingFile = null;
const filesIterator = storageFolder.getFilesByName(filename);
// ファイルが既に存在するかチェック
if (filesIterator.hasNext()) {
existingFile = filesIterator.next();
}
// ファイルが存在しない場合のみ保存
if (!existingFile) {
storageFolder.createFile(attachment).setName(filename);
}
}
}
使い方
GAS の使い方自体は他の記事を参照するとよいであろう。
追記(2024/01/27)
実は取得したいファイルが送信されてくるメーリスは、普段 Google Drive を使っている Google アカウントとは異なるアカウントであった。
GAS で異なるアカウントの Google Drive にアクセスするのはめんどくさい。
そこで、メーリスに入っているアカウントで、該当のメールが来たら Google Drive を使っている Google アカウントの Gmail に転送するようにした。
そして、GAS で Google Drive を使っている Google アカウントの Gmail に転送されたメールを処理するようにした。